Introduction
Hello! My name is Tan Mei Yen and I am currently a second year student studying Computer Science at National University of Singapore.
This Personal Project Portfolio details the contributions which I have made to the Equipment Manager project for CS2103T Software Engineering module. My main responsibilites are implementing command features which are filter
and sort
and also ensuring proper documentation in the user and developer guide.
The following sections illustrate these enhancements in further detail and other relevant sections I have added to the user and developer guides in relation to these enhancements.
Overview
Equipment Manager is a desktop CLI (Command Line Interface) application that allows engineers to keep track on the Preventive Maintenance schedule of all Resuscitation Devices in Singapore. It has a GUI (Graphic User Interface) that allows users to get further information on each equipment.
If you are an engineer that uses the computer frequently and want to improve efficiency at work, why not try out Equipment Manager? No installation is required!
Main Features of Equipment Manager:
-
Viewing of equipment information through typing
-
Searching and filtering by equipment serial number, equipment status or work list
-
Display equipment location in Google Maps
-
Plan the most efficient route to take for visiting multiple locations
Summary Of Contributions
This section is to provide a summary of my contributions to the project.
-
Enhancement 1: added the ability to filter the equipment list according to specified fields
-
What it does: The
filter
command allows the user to filter the equipment list which matches the given fields:-
Name
-
Address
-
Date
-
Phone
-
Tags
-
Serial Number
-
-
Justification: The user can filter the list with certain fields, expediting the search process, and reducing the time needed to find an equipment and its details.
-
Highlights:
-
The command is simple to use and allows multiple fields to filter by.
-
If users were to forget what are the fields available, error messages would guide them to execute the commands correctly.
-
-
Credits: Code from
FindCommand
was referenced in the implementation ofFilterCommand
-
-
Enhancement 2: added the ability to sort the equipment list according to specified fields
-
What it does: The
sort
command allows the user to sort the equipment list by the given fields:-
Name
-
Date
-
Serial Number
-
Phone
-
-
Justification: The user can sort the equipment list in lexicographical order for better viewing purposes.
-
Highlights:
-
The command is simple to use and allows the user to sort the list by their own preferences.
-
If users were to forget what are the fields available, error messages would guide them to execute the commands correctly.
-
-
-
Code contributed:
-
Click here to view my code on the CS2103T Project Code Dashboard.
-
-
Other contributions:
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide, which consists of documentation of filter and sort commands. They showcase my ability to write documentation targeting end-users.
Filtering the equipment list: filter
Filter the current shown list which match the given keywords.
Format: filter [n/NAME_KEYWORD]…[t/TAG_KEYWORD]…[a/ADDRESS_KEYWORD]
-
At least one keyword must be provided.
-
Filtering multiple keywords of the same prefix will return equipment whose attribute corresponding to the prefix contain any one of the keywords.
-
Filtering with keywords of different prefixes will return only equipment that matches with any of the keywords of the different prefixes.
-
The filter is case insensitive, e.g. hougang will match Hougang.
-
The user can filter the equipment list with any specified fields, and can filter by multiple fields and keywords.
This command only works on the overall equipment list and not the current shown equipment list. |
For example,
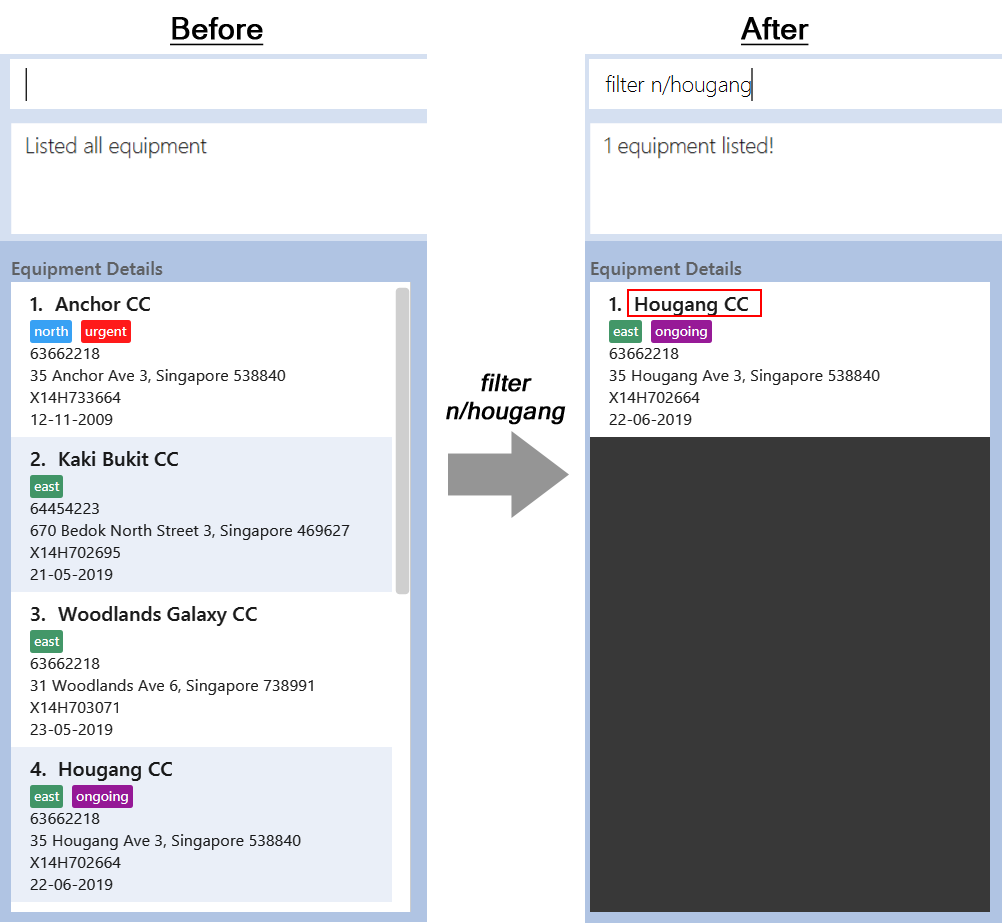
-
filter n/hougang
Returns any equipment whose name consists of 'hougang'.
Sorting the equipment list: sort
Sort the equipment list.
Format: sort [FIELD_NAME to be sorted by]
The user can sort the equipment list with specified field.
The sort parameters are case-insensitive.
By default, sort
sorts the list by name in lexicographical order.
Equipment list can only be sorted by name , date , phone and serial .
|
For example,
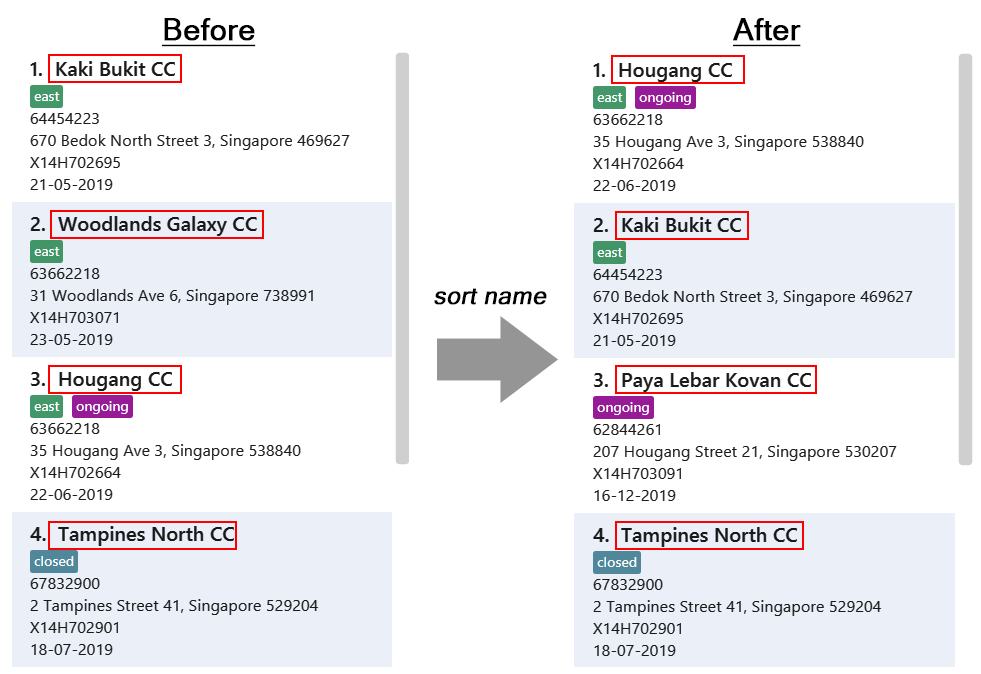
-
sort name
Returns the list sorted in alphabetical order of the client’s name.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide, which consists of documentation of filter and sort commands and user stories. They showcase my ability to write technical documentation and the technical depth of my contributions to the project.
Filter feature
Introduction
We have implemented a FilterCommand
that allow users to filter the equipment list with the specified fields.
The filter feature allow users to filter the equipment list with any specified fields, and also can filter by multiple fields.
The FilterCommand
is able to filter the equipment list according to the user’s preference at a time.
Current Implementation
The filter mechanism is supported by FilterCommandParser
. It implements Parser
that implements the following operation:
-
FilterCommandParser#parse()
- Checks the arguments for empty strings and throws a ParseException if empty string is found. It then splits the arguments usingArgumentTokenizer#tokenize()
and returns an ArgumentMultimap. Keywords of the same prefix are then grouped usingArgumentMultimap#getAllValues()
.
The filter mechanism is also facilitated by FilterCommand
. It extends Command
and implements the following operation:
FilterCommand#execute()
— Executes the command by updating the current FilteredPersonList
with the EquipmentContainsKeywordPredicate
.
EquipmentContainsKeywordsPredicate
takes in the lists of keywords for the following:
-
Name
-
Address
-
Date
-
Phone
-
Tags
-
Serial Number
The following sequence diagram shows how the filter operation works:
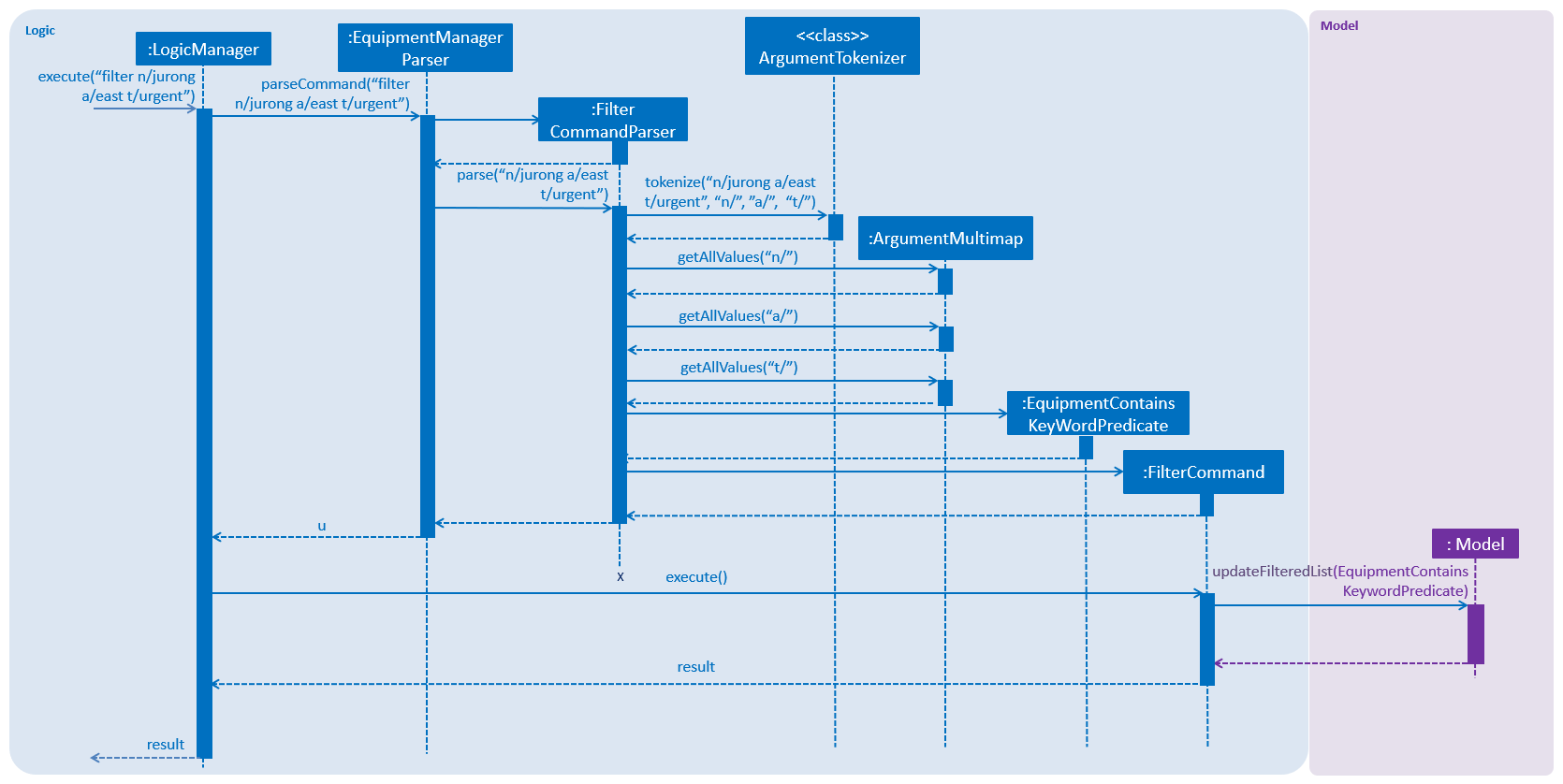
Example
Given below is an example usage scenario of how the filter mechanism behaves at each step when filtering.
Step 1. The user launches the application.
Step 2. The user executes filter n/jurong a/west t/urgent
command to get all fields whose equipment contains the keywords
Step 3. After EquipmentManagerParser
detects filter as the command word, a FilterCommandParser#parse()
is called and
the EquipmentContainsKeywordsPredicate is constructed with the arguments of the filter command.
Step 4. FilterCommand#execute()
is then called.
Step 5. The entire equipment list is filtered by the predicate EquipmentContainsKeywordsPredicate
.
Step 6. Then, EquipmentContainsKeywordsPredicate
checks that the Equipment Manager has either the respective
attributes - serial number, tags, address, name, preventive maintenance date, phone.
Step 7. The argument is filtered against the predicate and returned to the GUI.
FilterCommand only filters the equipment list.
|
Design Considerations
Implementation of FilterCommand
-
Alternative 1 (current choice): Require user to prepend every keyword argument with the appropriate attribute prefix. Supports multiple fields in the same command.
-
Pros: It is easy to implement and easy to match keyword against an equipment if the matching attribute is known.
-
Pros: User has more control over the results returned.
-
Pros: User can also filter by multiple fields. e.g:
filter n/jurong t/west
-
Cons: User is required to type slightly more.
-
Cons: It only filters the equipment list.
-
-
Alternative 2: filter by specific fields
-
Pros: It is easy to implement and it is also consistent with how
FilterCommand
works. -
Cons: User has less control over the results returned.
-
Cons: User can input anything and the results returned is not specific by type.
-
-
Alternative 3: filter by tags
-
Pros: It is more specific and more restricted.
-
Cons: More difficult to implement
-
Cons: Too restricted as it is only filtered by tags.
-
Sort feature
Introduction
We have implemented a SortCommand
that allow users to sort the equipment list with specific field.
The entries in the equipment list is ordered to the time when the entry is entered into the application by default such that the entry entered first is at the top of the equipment list and the latest entry entered is at the bottom of the equipment list.
The sort
mechanism allows user to view the equipment list according to their preferences.
The SortCommand
is able to sort the equipment list according to the user’s preferences at a time.
Current Implementation
The sort
command sorts the list by specified field in lexicographical order.
Comparators that implement java.util.Comparator
interface are used in the sort mechanism to perform the comparsion.
The sort mechanism is supported by SortCommandParser
. It implements Parser
that implements the following operation:
-
SortCommandParser#parse()
- Checks the arguments for empty strings and throws a ParseException if empty string is found. It then splits the arguments and checks if the next string is a valid field, else, it will throw a ParseException.
Valid fields:
-
name
-
date
-
phone
-
serial
The following sequence diagram shows how the sort operation works:
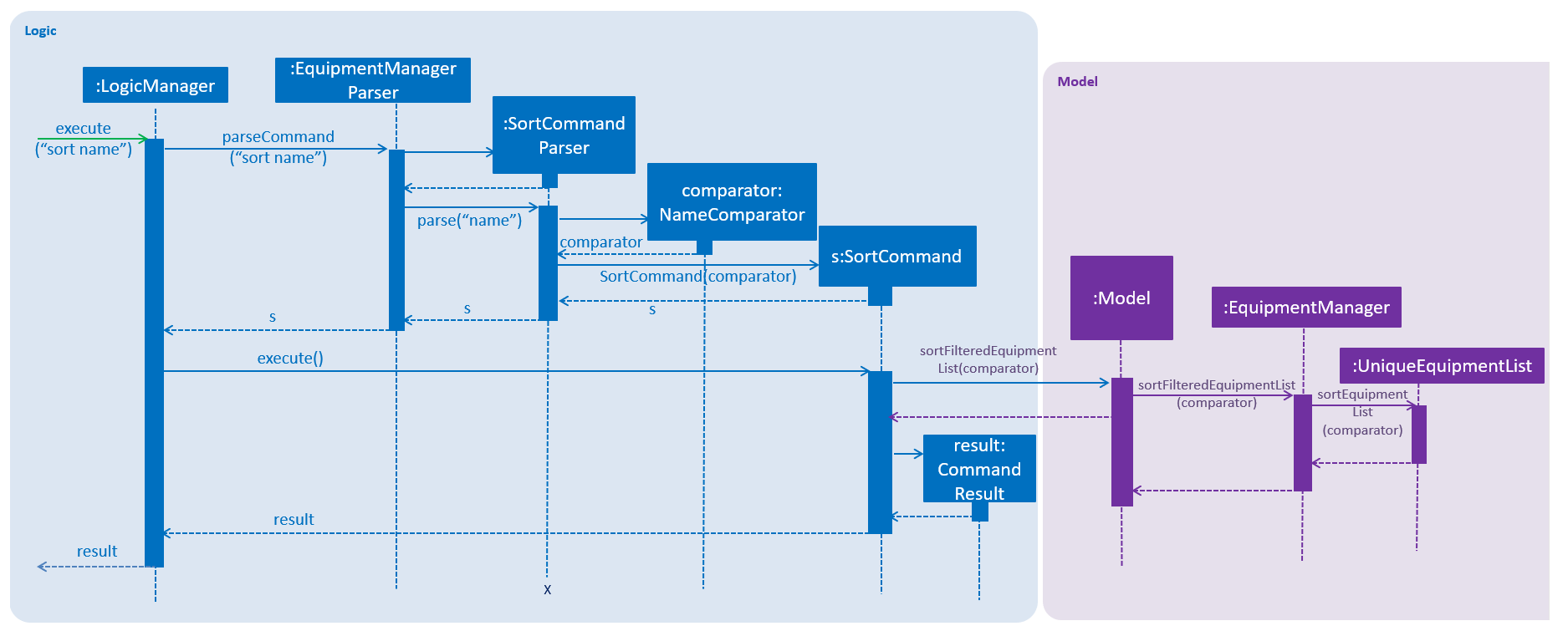
Example
Given below is an example usage scenario of how the sort mechanism behaves at each step when sorting.
Step 1. The user launches the application.
Step 2. The user executes sort name
command to sort the equipment list by name.
Step 3. SortCommandParser#parse()
creates a new NameComparator()
object and passes it into SortCommand
.
Step 4. EquipmentManager#sortEquipmentList(comparator)
calls UniqueEquipmentList#sortEquipmentList(comparator)
, which then
uses FXCollection’s static method sort()
to sort the equipment list by name.
Step 5. The list is sorted by specified field (name) and returned to the GUI.
Test cases:
-
Input:
sort
Output: An error message will be displayed to show what are the fields available.
-
Input:
sort name
Output: The list is sorted by the name in alphabetical order.
-
Input:
sort date
Output: The list is sorted in ascending order by the the preventative maintenance date of the equipment.
-
Input:
sort phone
Output: The list is sorted in ascending order by the phone number of the client.
-
Input:
sort serial
Output: The list is sorted in ascending order by the serial number of the equipment.
SortCommand only sorts the equipment list.
|
Design Considerations
Implementation of SortCommand
-
Alternative 1 (current choice): Sorts by specific field by using the Comparator interface.
-
Pros: Sorting can be done based on different fields (name, date, phone number and serial number)
-
Cons: A new class that implements the interface Comparator needs to be created for the fields.
-
-
Alternative 2: Sort by client name
-
Pros: Overall list is sorted fully by client name
-
Cons: Unable to sort other specific fields such as serial number of the equipment.
-